POSTS
- 3 minutes readFlutter is a free and open-source mobile UI framework that has been gaining a lot of popularity. It is created by Google and was unveiled at the 2015 Dart developer summit. Some of the advantages of using Flutter are:
-
Build cross-platform apps - It allows the building of cross-platform apps from a single code base. This means you can now target Android and iOS using a single codebase.
-
Hot reload - The Hot reload feature allows you to quickly reload the code on a running app as and when you make code changes.
-
Faster rendering - Flutter uses the Skia library, the same rendering library as the Chrome browser which makes rendering faster.
Flutter also provides support for web applications. You can read more about that here
In this post, we will see how we can use our Headless CMS to power the contents of your mobile apps. This means you don’t need any database or custom solutions to manage content.
To get started, we will first need to import the Dialoguewise package like this:
import 'package:dialogue_wise/dialogue_wise.dart';
Next, we need to prepare the payload and its pretty straight forward.
var request = new DialogueWiseRequest();
request.slug = '[Your Slug]';
request.apiKey = '[Your API Key]';
request.emailHash='[The hash of your email]';
Now that you have the payload ready, all you need to do is to call the service with the payload.
var dialogueWiseService = new DialogueWiseService(new http.Client());
Map response = await dialogueWiseService.getDialogue(request);
You can now run the command pub dev to resolve all the dependencies and then run dart main.dart where main.dart is the file containing your code.
That’s it! The contents are now available in the response variable and you just need to display it on your app. You can use a Widget
control to display it and you should be able to see something like this.
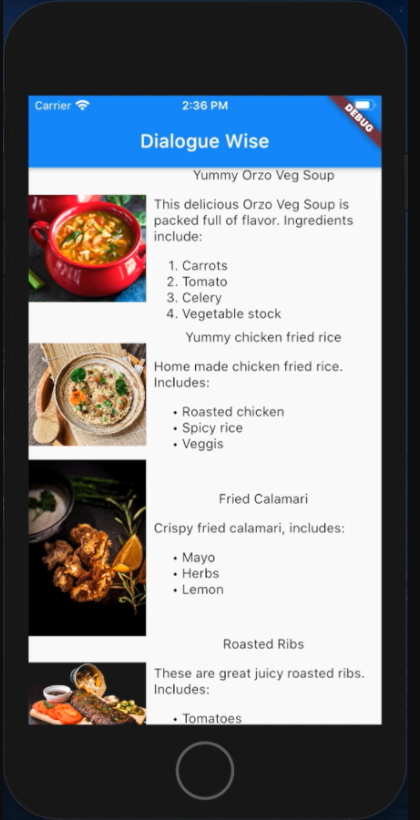
The entire source code is available on Github. Also, check out our Docs to understand how you can make use of other features.
For step by step instructions, you can also checkout our Youtube video below:
Also, feel free to check out our Flutter Web project.
As you can see the integration is pretty much straightforward. Some of the advantages of using our platform are:
-
Location / Device based content delivery - Allows you to enhance the user experience by automatically delivering content based on the user’s location or device.
-
Custom scripting - Add custom scripts to further personalize the user experience based on your predetermined scenarios.
-
Webhooks - Get real-time notifications whenever your content gets changed. This allows you to do things like, trigger a build or fetch updated content.